Harsha's blog
Wednesday, April 9, 2014
How to configure RabbitMQ to use more memory and disk space
Sunday, December 22, 2013
How to create your own Maven Repository in Git hub
Clone the empty repository to your home directory or what ever location you prefer.
git clone https://github.com/Harsha/maven-repo.git maven-repo
my local repository path is /home/harsha/maven-repo
Inside your project which you would like to share jar files in maven-repo define maven deployment plugin as below in pom.xml.
<distributionManagement>
<repository>
<id>internal.repo</id>
<name>Internal Repository</name>
<url>${internal.repo.path}</url>
</repository>
</distributionManagement>
<internal.repo.path>
which should points to your local repository which you get cloned above. <groupId>com.harsha.company.project</groupId>
<artifactId>module-name</artifactId>
<packaging>jar</packaging>
<version>1.0</version>
<properties>
<internal.repo.path>file:/home/harsha/maven-repo/</internal.repo.path>
</properties>
mvn deploy
inside your project. It will create all the artifacts and publish to the local maven repository which you get cloned above.Now you can see all the artifacts are published in your local repository - /home/harsha/maven-repo. Just commit these changes and pushed to the git-hub.
How to download published jars from the repository.
Use below repository to download dependencies from your repository.
<repositories>
<repository>
<id>maven-repo</id>
<url>https://github.com/Harsha/maven-repo/raw/master</url>
</repository>
</repositories>
<dependency>
<groupId>com.harsha.company.project</groupId>
<artifactId>module-name</artifactId>
<version>1.0</version>
</dependency>
Saturday, June 13, 2009
How to access Axis IP Camera using Java
What is an IP Camera?
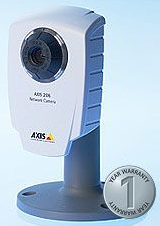
This was indeed a challenge for me at the first time. But with mere investigation i could find that before accessing the IP camera we need to obtain authentication as it is working as a video server. I would list out what i did to capture a video stream from IP camera
Step1 : obtaining the IP address of the camera (it will get assigned an available ip address from the network as it is configured upon DHCP by default) . You can use the utility software provided by the camera provider in case of finding the ip.
Step2 : You may need to configure the axis camera giving a password for the default "root" user. If you want you may add many users as you wish using their utility software.
Step3 : Then you can go to the URL of the camera and make sure the JPG or MJPG streams are there.
Step4 : Next challenge was to obain authentication from the video server of the camera using Java code snippet. In that case i used java.net.Authenticator. Authenticator would consist of the URL , username and password which we are providing for the server.Then
setDefault(Authenticator a)
Sets the authenticator that will be used by the networking code when a proxy or an HTTP server asks for authentication.Step5 : Now you are successfully connected to the Camera. :)
Step6 : Then you can use the video stream from the IP camera for whatever application you use.
Tuesday, May 19, 2009
How to configure a Star Printer (TSP 800)
The TSP800 is the only printer on the market that provides printing on 3” to 4.4” wide paper at an amazing speed. It is the perfect printer for applications that need to print a large amount of information on a receipt
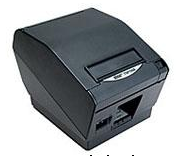
1) First identify the interface of the printer, my one is having Eathernet Interface.
2) Accordingly you may guess how you would connect your printer. e.g : If it is having an USB interface it may be directly connected to the computer. If Eathernet interface it would be connected to a Network add as a Network printer (TCP/IP).
Below i asssume the printer as TSP800 Star Printer having Eathernet Interface.
3) Before adding your printer as a network printer you have to find out the IP address of the printer. Most of the printers at the first time are configured upon DHCP , so it may assign an IP for itself dynamically. For proper and ease of use you have to assign a static IP for the printer.
4) In case of finding the dynamically assigned IP address of the printer , the only way you have to follow is printing the self test page which would contain each and every information of the printer statistics. (Note: when you take a self test page just press the feed button once just as you turn on the printer, do not press consequently as it may lead to some problems. While printing it may suspend in the middle, do not think it as a problem, it is because of the time taken to identify information of the network card in built to the printer)
5) Now you can go to the printer interface (web page) using the IP found in the test page. (http://ip of the printer) . This will led to access the system access interface of the printer in case of assigning a static IP
6) Use defauld username and password as in your test page. otherwise (root , public). This would carry you to the system access interface. There you can assign a static IP to the printer.
7) You are almost done : Now add the printer as a TCP/IP printer.How to add your printer as a TCP/IP printer is listed here http://www.tamu-commerce.edu/ctis/help/tcpipprinter/default.htm. Note: use the ip address you assigned in step 8. Port would be assigned automatically.
8) Print a test page to test your printer. You are done :)
Saturday, March 29, 2008
Google Summer of Code 2008 Proposal
Subject | Review Application |
Author | Harshajith Halgaswatta |
|
Project Title
Review Application
Abstract
XWiki is a generic wiki platform allowing the development of collaborative applications. It includes a toolkit for the web, supporting a cost-effective solution that allows non-developers to create those required applications quickly and in an organic manner. If one application remain as it is from the orientation and no way to give feed backs regarding to those documents or comments on it, its worth and usability may degrade because it is not updating in a regular manner.
Hence the intention of this project is to give the facility to add reviews (add comments, highlight text, delete text, insert text, with an interface like the one in Adobe Acrobat Professional). Further it gives a way to anchoring reviews without messing with previous reviews. Eventually the authors of document may do required changes according to the reviews.
Deliverables
1. Implementation of Review Application (Client portion and author’s portion)
2. Tests for Review Application according to its adjectives
3. Documentation to support continuation of the project
Overview
People once read it and move off if it contain some useless things or it does not include what essentially should be there. Therefore if there is a way to update documents and particular stuff in an easy way according to the reviews of web viewers it will help the authors and the proprietors to remain their web viewers in a stable level.
Therefore this project supposes to provide a review application which leads to add reviews just by clicking on the particular spot of a page and add their reviews. It may be a cumulative or standalone mode. Further it may beneficial giving the chances to view reviews not only by the document author but also the other viewers. Eventually it support a vital pros in point of authors view that they are in a position to edit, delete , remove highlights and comments of reviewers and make changes appropriately just by clicking once.
I hope to develop this project dividing into two modules.
First module is the client portion which is indeed giving the facility to add reviews (feedback or comment on the read only document) for a particular document. Also it will support a way for the reviewed data to be anchored, so that later edits won't mess all the reviews.
Second module is the Author’s portion .The author can view such a review (or all of them together) and:
easily apply "delete" and "insert" changes with one click.
easily remove the comments and highlights.
while being able to also edit the document.
Project Plan
I have broken down the project under 4 steps as follows.
Step1: Initial Planning and Designing
I would look into the current implementation of Xwiki under this step(velocity and groovy). It would be very important to plan and design how I reach towards predefined objectives. Eventually I would have the skeleton of the implementation.
Estimated Completion: 26th May 2008
Step2: Implementation
The real logic for client and author portion of review application would take into accounts and starts implementation.
Deliverable(s): Prototype and documentation for mid evaluation Estimated Completion: 2nd July 2008
Step3: Modifications and Tests
Modifications or improvements suggested at the mid evaluation would be completed in this step.
Deliverable(s): Prototype including tests Estimated Completion: 6th August 2008
Step4: Final Product and Documents
This step would complete the Review Application. Necessary documents would also be present with the final product.
Deliverable(s): Final product and documentation Estimated Completion: 1st September 2008.
Biography
I’m a level 3 undergraduate of the Department of Computer Science & Engineering of the University of Moratuwa, Sri Lanka. I have participated in some Open Source development.
During my internship I have implemented a member section from the scratch for E-channeling private Ltd, which is the market leader of online channel booking system and first listed company in Colombo stock exchange in Sri Lanka. I implemented the whole member section which included member usage module and member administration module using java upon STRUTS2 framework with Sun Application Server and ORACLE database server. Further I used some XML stuff in case of merchant integration for the site.
I have implemented a managements system for an Internet cafe as my level 3 programming project module at the university. It covered many areas of JAVA (Swing toolkit, Java Network programming, jdic integration, java and XML, Java mail API).I used MySql server in case of this project.
I’m really passionate in java and some web frameworks such as Struts2 and Spring. Thus I found this project really appealing as it lies on the path of my experience. I believe that I have the necessary background knowledge to make this a success. Eventually I hope that my involvement in this project would polish my software engineering skills whilst adding a whole new experience to my career.
Mentor Information
Sergiu Dumitriu, Marta Girdea
References
http://www. xwiki/article.tss.html
http://www. Xwiki/SecondGenerationWiki.htm